Web server is a place which stores, processes and delivers web pages to Web clients. Web client is nothing but a web browser on our laptops and smartphones. The communication between client and server takes place using a special protocol called Hypertext Transfer Protocol (HTTP).
In this protocol, a client initiates communication by making a request for a specific web page using HTTP and the server responds with the content of that web page or an error message if unable to do so (like famous 404 Error). Pages delivered by a server are mostly HTML documents.
ESP8266 Operating Modes
One of the greatest features ESP8266 provides is that it cannot only connect to an existing WiFi network and act as a Web Server, but it can also set up a network of its own, allowing other devices to connect directly to it and access web pages. This is possible because ESP8266 can operate in three different modes:
Station mode,
Soft Access Point mode,
and both at the same time.
Station mode
The ESP8266 that connects to an existing WiFi network (one created by your wireless router) is called Station(STA), In STA mode ESP8266 gets IP from wireless router to which it is connected. With this IP address, it can set up a web server and deliver web pages to all connected devices under existing WiFi network.
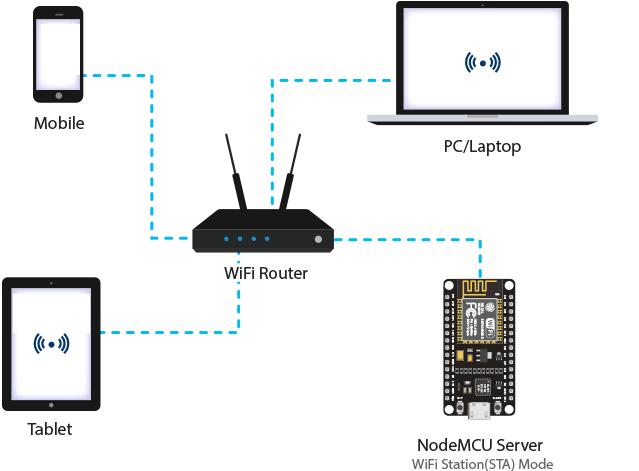
Soft Access Point Mode
The ESP8266 that creates its own WiFi network and acts as a hub (Just like WiFi router) for one or more stations is called Access Point(AP). Unlike WiFi router, it does not have interface to a wired network. So, such mode of operation is called Soft Access Point(soft-AP). Also the maximum number of stations that can connect to it is limited to five.
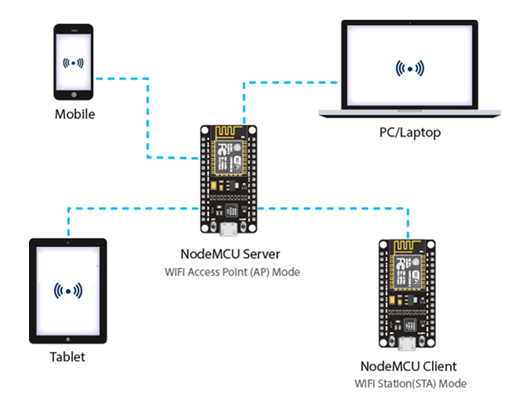
Web servers
A web server is an Internet-connected device that stores and serves files. Clients can request such a file or another piece of data, and the server will then send the right data/files back to the client. Requests are made using HTTP.
HTTP
HTTP or the Hypertext Transfer Protocol is the text-based protocol used to communicate with (web) servers. There are multiple HTTP request methods, but I'll only cover the two most widely used ones: GET and POST.
HTTP GET
GET requests are used to retrieve data from a server, a web page for instance. It shouldn't change anything on the server, it just gets the data from the server, without side effects.
For example : Using your mobile phone to access a website (URL) like www.watchitgroup.online
When you open a webpage in your browser, it will take the URL and put it in an HTTP GET request. This is just plain text. Then it will send the request to the right server using TCP(TCP/IPstands for Transmission ControlProtocol/InternetProtocol, which is a set of networking protocols that allows two or more computers to communicate). The server will read the request, check the URL, and send the right HTTP response for that URL back to the browser.
HTTP POST
POST requests are used to send data to the server, for example, to send your user name and password to the server when you log in, or when you upload a photo. Unlike GET, POST can change the data on the server or the state of the server. POST has a body that can contain data that is sent to the server.
HTTP status codes
A server should answer all requests with an HTTP status code. This is a 3-digit number indicating if the request was successful or telling the client what went wrong. Here's a table with some of the most important and useful ones.
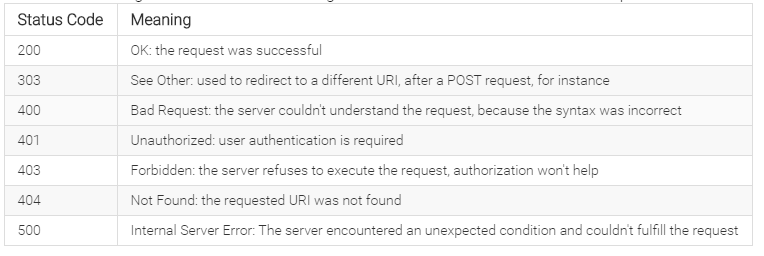
Simple Webserver (HelloWorldWebserver)
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266WebServer.h>
const char* ssid = "Arsy"; // SSID use the name of your network
const char* password = "1234567l"; // password of your network
ESP8266WebServer server(80); // port of the server
void setup(void)
{
Serial.begin(115200);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
Serial.println("");
// Wait for connection
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
server.on("/", handleRoot);
server.on("/inline", []() {
server.send(200, "text/plain", "this works as well");
});
server.onNotFound(handleNotFound);
server.begin();
Serial.println("HTTP server started");
}
void loop(void)
{
server.handleClient();
}
void handleRoot()
{
server.send(200, "text/plain", "hello from esp8266!");
}
void handleNotFound()
{
String message = "File Not Found\n\n";
message += "URI: ";
message += server.uri();
message += "\nMethod: ";
message += (server.method() == HTTP_GET) ? "GET" : "POST";
message += "\nArguments: ";
message += server.args();
message += "\n";
for (uint8_t i = 0; i < server.args(); i++) {
message += " " + server.argName(i) + ": " + server.arg(i) + "\n";
}
server.send(404, "text/plain", message);
}
Code Explanation
Include library of the esp8266 board
Setup your network credentials (SSID & password) with your own network name and password
const char* ssid = "Arsy";
const char* password = "1234567l";
Webserver ibject that listens for http request on port 80 meaning your IPaddress :80 , for exampe 172.20.10.03 :80 , but 80 is by default you don’t need to put it unless you change it in the code
ESP8266WebServer server(80); // port of the server
in the setup function , there is defining the wifi mode (Stationary as web server), also to check if the network is connected, if network is not connected you will see the dots ..... being printed in the Serial Monitor
void setup(void)
{
Serial.begin(115200);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
Serial.println("");
// Wait for connection
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Tell us what network we're connected to and Send the IP address of the ESP8266 to the computer Serial monitor
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);// network name
Serial.print("IP address: ");
Serial.println(WiFi.localIP()); // IP address
Call the 'handleRoot' function when a client requests URI "/"
server.on("/", handleRoot);
your Esp8266 IP address /inline (and click enter ) , "this works as well" will be printed meaning this is another roots. for example : 172.20.10.03 /inline (click enter) ,
server.on("/inline", []() {
server.send(200, "text/plain", "this works as well");
// When a client requests an unknown URI (i.e. something other than "/"), call function "handleNotFound"
server.onNotFound(handleNotFound);
Listen for HTTP requests from clients
void loop(void)
{
server.handleClient();
}
Send HTTP status 200 (Ok) and send some text to the browser/client
void handleRoot()
{
server.send(200, "text/plain", "hello from esp8266!");
}
Send HTTP status 404 (Not Found) when there's no handler for the URI in the request
void handleNotFound()
{
String message = "File Not Found\n\n";
message += "URI: ";
message += server.uri();
message += "\nMethod: ";
message += (server.method() == HTTP_GET) ? "GET" : "POST";
message += "\nArguments: ";
message += server.args();
message += "\n";
for (uint8_t i = 0; i < server.args(); i++) {
message += " " + server.argName(i) + ": " + server.arg(i) + "\n";
}
server.send(404, "text/plain", message);
}
OutPut in the Serial Monitor
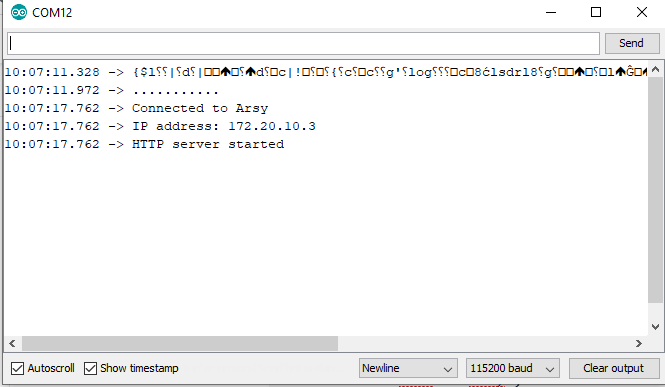
copy the Ip address in the browser , you should be able to see this .
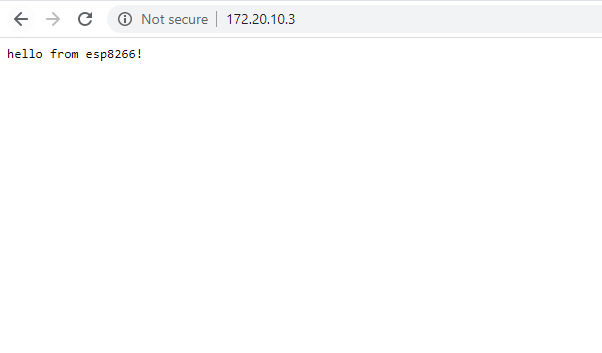
also try , 172.20.10.3/inline , this also will print the following , this was enabled by these code server.send(200, "text/plain", "this works as well");
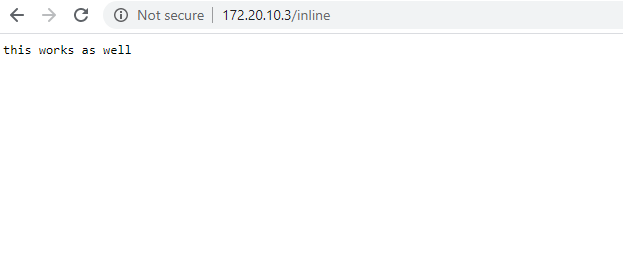
play with these code , you will be ready to understand more about these following code.
ESP8266 LED webserver Control an LED
IoT based LED control from web page using ESP8266. This is actually the beginning for home automation project .
To control the LED using Web server we need to create an HTML web page. The page will have two buttons for turning LED ON and OFF.
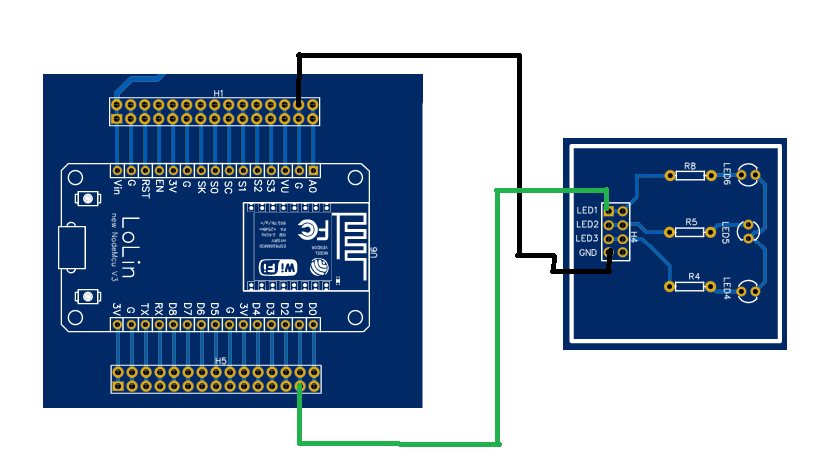
circuit diagram esp8266 and LED circuit circuit section
Expected result
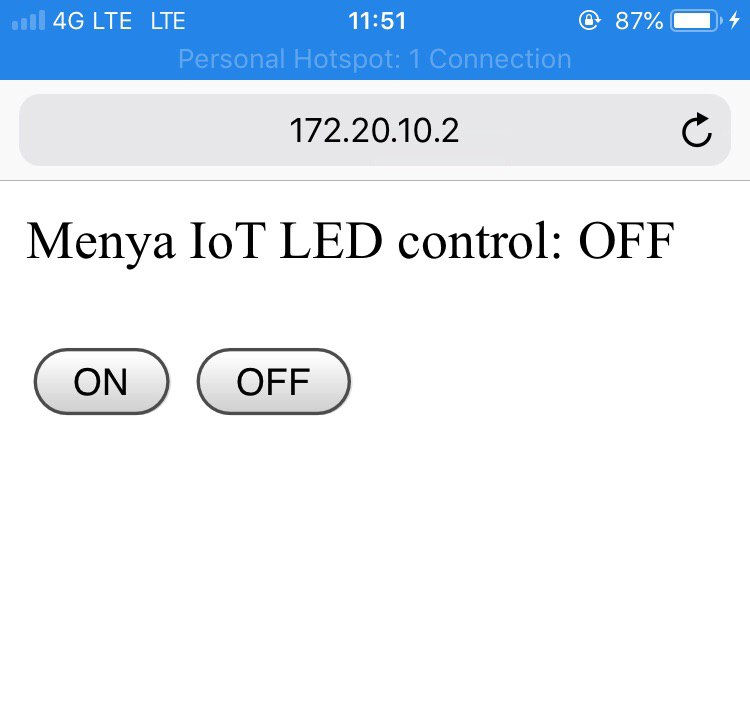
Web page control LED.
Sketch
// The first thing you need to do is include the ESP8266 WiFi library.
#include <ESP8266WiFi.h>
// Your network credentials
const char* ssid = "Arsy"; // Your Wi-Fi Name
const char* password = "1234567l"; // Wi-Fi Password
// GPIO 5 meaning D1 pins
int LED = 5;
// Set web server port number to 80 , 80 port number is known as default port
WiFiServer server(80);
void setup()
{
Serial.begin(115200); //Default Baudrate
pinMode(LED, OUTPUT);
digitalWrite(LED, LOW);
Serial.print("Connecting to the Newtork");
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED)
{
delay(500);
Serial.print(".");
}
Serial.println("WiFi connected");
server.begin(); // Starts the Server
Serial.println("Server started");
Serial.print("IP Address of network: "); // will IP address on Serial Monitor
Serial.println(WiFi.localIP());
Serial.print("Copy and paste the following URL: https://"); // Will print IP address in URL format
Serial.print(WiFi.localIP());
Serial.println("/");
}
void loop()
{
WiFiClient client = server.available();
if (!client)
{
return;
}
Serial.println("Waiting for new client");
while(!client.available())
{
delay(1);
}
String request = client.readStringUntil('\r');
Serial.println(request);
client.flush();
int value = LOW;
if(request.indexOf("/LED=ON") != -1)
{
digitalWrite(LED, HIGH); // Turn LED ON
value = HIGH;
}
if(request.indexOf("/LED=OFF") != -1)
{
digitalWrite(LED, LOW); // Turn LED OFF
value = LOW;
}
//*------------------HTML Page Code---------------------*//
client.println("HTTP/1.1 200 OK"); //
client.println("Content-Type: text/html");
client.println("");
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.print(" Menya IoT LED control: ");
if(value == HIGH)
{
client.print("ON");
}
else
{
client.print("OFF");
}
client.println("<br><br>");
client.println("<a href=\"/LED=ON\"\"><button>ON</button></a>");
client.println("<a href=\"/LED=OFF\"\"><button>OFF</button></a><br />");
client.println("</html>");
delay(1);
Serial.println("Client disonnected");
Serial.println("");
}
Video
you can see that the web design is very basic , but there is also a possibility to use bootstrap web technology to have good looking web page. We will see it in the next Tutorials.
Let us know in the comment section if it worked 👌!
can someone help me to solve this error
Sketch uses 274928 bytes (26%) of program storage space. Maximum is 1044464 bytes.
Global variables use 27200 bytes (33%) of dynamic memory, leaving 54720 bytes for local variables. Maximum is 81920 bytes.
esptool.py v2.6
2.6
esptool.py v2.6
Serial port COM7
Connecting....
Chip is ESP8266EX
Features: WiFi
MAC: fc:f5:c4:8b:e8:91
Uploading stub...
Running stub...
Stub running...
Configuring flash size...
Auto-detected Flash size: 4MB
Compressed 279088 bytes to 201415...
Wrote 279088 bytes (201415 compressed) at 0x00000000 in 18.0 seconds (effective 124.2 kbit/s)...
Hash of data verified.
Leaving...
Hard resetting via RTS pin...
hey everyone how can I get this error solved
xecutable segment sizes:
IROM : 244616 - code in flash (default or ICACHE_FLASH_ATTR)
IRAM : 27292 / 32768 - code in IRAM (ICACHE_RAM_ATTR, ISRs...)
DATA : 1264 ) - initialized variables (global, static) in RAM/HEAP
RODATA : 1132 ) / 81920 - constants (global, static) in RAM/HEAP
BSS : 25032 ) - zeroed variables (global, static) in RAM/HEAP
Sketch uses 274304 bytes (26%) of program storage space. Maximum is 1044464 bytes.
Global variables use 27428 bytes (33%) of dynamic memory, leaving 54492 bytes for local variables. Maximum is 81920 bytes.
in compilling
THEN
xecutable segment sizes:
IROM : 244616 - code in flash (default or ICACHE_FLASH_ATTR)
IRAM : 27292 / 3…
it worked thank you guys for your help
can you kindly please explain step by step what to do after opening the serial monitor
Open the Serial Monitor , as per the video